Read In From File C++

Reading data from a file is a fundamental task in programming, and C++ provides several methods to accomplish this. In this blog post, we will explore different techniques to read data from a file, focusing on the various approaches, their advantages, and how to implement them effectively. Whether you're a beginner or an experienced programmer, understanding these methods will enhance your file handling skills in C++.
Using the Standard Library: ifstream

The Standard Library in C++ offers a straightforward way to read data from files using the ifstream
class. This class is part of the fstream
library, which stands for "file stream." Here's a step-by-step guide on how to use ifstream
to read data from a file:
Step 1: Include the Necessary Header

First, you need to include the fstream
header in your C++ code. This header provides the necessary definitions and declarations for file handling:
#include
Step 2: Create an ifstream Object

Next, you'll create an ifstream
object. This object represents the file you want to read from. You can choose a variable name that makes sense for your context. Here's an example:
ifstream inputFile;
Step 3: Open the File
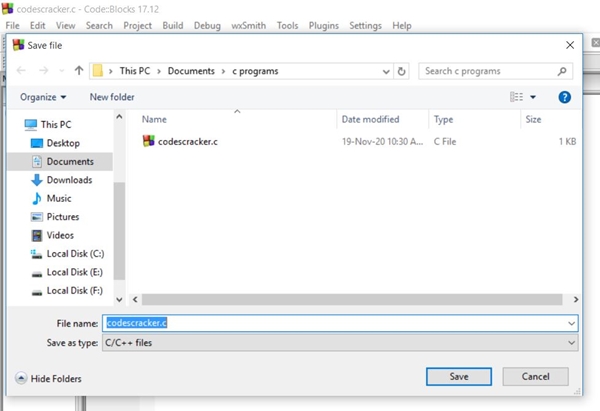
Use the open
function to specify the file you want to read. You can provide the file path as an argument. Here's how you can open a file named "data.txt" in the current directory:
inputFile.open("data.txt");
Step 4: Check if the File is Open

It's crucial to check if the file was successfully opened. You can use the is_open
function to verify this. If the file fails to open, you should handle the error appropriately. Here's an example:
if (!inputFile.is_open()) {
// Handle the error, e.g., print an error message
cerr << "Failed to open the file." << endl;
return;
}
Step 5: Read Data from the File

Once the file is open, you can read data from it using various methods. Here are a few common approaches:
Reading Line by Line
To read data line by line, you can use a loop and the getline
function. This function reads a line of text from the file and stores it in a string. Here's an example:
string line;
while (getline(inputFile, line)) {
// Process the line of data
// ...
}
Reading Individual Characters
If you need to read individual characters from the file, you can use the get
function. This function reads a single character and stores it in a variable. Here's an example:
char character;
while (inputFile.get(character)) {
// Process the character
// ...
}
Reading Specific Data Types
To read specific data types like integers, floats, or doubles, you can use the stream insertion operator (>>
). This operator reads data from the file and extracts it into the desired data type. Here's an example for reading integers:
int number;
while (inputFile >> number) {
// Process the integer
// ...
}
inputFile.clear(); // Clear the EOF flag
inputFile.ignore(); // Ignore the newline character
Step 6: Close the File

After you're done reading data from the file, it's essential to close it properly. You can use the close
function to do this. Here's an example:
inputFile.close();
Reading Data with File I/O Functions

In addition to the ifstream
class, C++ provides a set of standard library functions for file input and output (I/O). These functions are part of the cstdio
library and offer a more low-level approach to file handling. Here's how you can use these functions to read data from a file:
Step 1: Include the Necessary Header

First, you need to include the cstdio
header in your C++ code. This header provides the necessary definitions and declarations for file I/O functions:
#include
Step 2: Open the File

Use the fopen
function to open the file for reading. This function takes two arguments: the file path and the mode. The mode specifies how you want to access the file. For reading, you can use the "r" mode. Here's an example:
FILE* file = fopen("data.txt", "r");
Step 3: Check if the File is Open
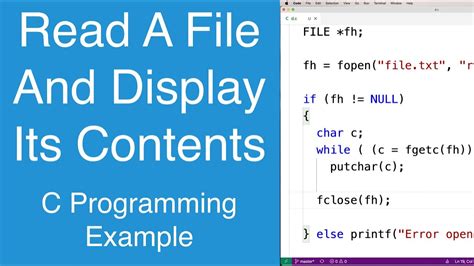
Similar to the ifstream
approach, you should check if the file was successfully opened. You can use the ferror
function to verify this. If the file fails to open, you should handle the error appropriately. Here's an example:
if (ferror(file)) {
// Handle the error, e.g., print an error message
printf("Failed to open the file.\n");
fclose(file); // Close the file if it failed to open
return;
}
Step 4: Read Data from the File

Once the file is open, you can read data from it using various functions. Here are a few common approaches:
Reading Line by Line
To read data line by line, you can use the fgets
function. This function reads a line of text from the file and stores it in a string. Here's an example:
char line[1000];
while (fgets(line, sizeof(line), file)) {
// Process the line of data
// ...
}
Reading Individual Characters
If you need to read individual characters from the file, you can use the fgetc
function. This function reads a single character and returns it as an integer. Here's an example:
int character;
while ((character = fgetc(file)) != EOF) {
// Process the character
// ...
}
Reading Specific Data Types
To read specific data types like integers, floats, or doubles, you can use the fscanf
function. This function reads data from the file and extracts it into the desired data type. Here's an example for reading integers:
int number;
while (fscanf(file, "%d", &number) == 1) {
// Process the integer
// ...
}
Step 5: Close the File
After you're done reading data from the file, it's essential to close it properly. You can use the fclose
function to do this. Here's an example:
fclose(file);
Reading Data with C++ Streams

C++ also provides a stream-based approach to reading data from files. This method is similar to the ifstream
class but uses the standard stream library. Here's how you can use C++ streams to read data from a file:
Step 1: Include the Necessary Header
First, you need to include the iostream
header in your C++ code. This header provides the necessary definitions and declarations for stream-based I/O:
#include
Step 2: Open the File
Use the ifstream
class to open the file for reading. This class is similar to the one we used earlier, but it's part of the stream library. Here's an example:
ifstream inputFile("data.txt");
Step 3: Check if the File is Open
Similar to the previous methods, you should check if the file was successfully opened. You can use the is_open
function to verify this. If the file fails to open, you should handle the error appropriately. Here's an example:
if (!inputFile.is_open()) {
// Handle the error, e.g., print an error message
cerr << "Failed to open the file." << endl;
return;
}
Step 4: Read Data from the File
Once the file is open, you can read data from it using stream operators. Here are a few common approaches:
Reading Line by Line
To read data line by line, you can use a loop and the stream extraction operator (<<
). This operator reads a line of text from the file and stores it in a string. Here's an example:
string line;
while (getline(inputFile, line)) {
// Process the line of data
// ...
}
Reading Individual Characters
If you need to read individual characters from the file, you can use the stream extraction operator (<<
). This operator reads a single character and stores it in a variable. Here's an example:
char character;
while (inputFile >> character) {
// Process the character
// ...
}
Reading Specific Data Types
To read specific data types like integers, floats, or doubles, you can use the stream extraction operator (<<
). This operator reads data from the file and extracts it into the desired data type. Here's an example for reading integers:
int number;
while (inputFile >> number) {
// Process the integer
// ...
}
Step 5: Close the File
After you're done reading data from the file, it's essential to close it properly. You can use the close
function to do this. Here's an example:
inputFile.close();
Handling File Errors
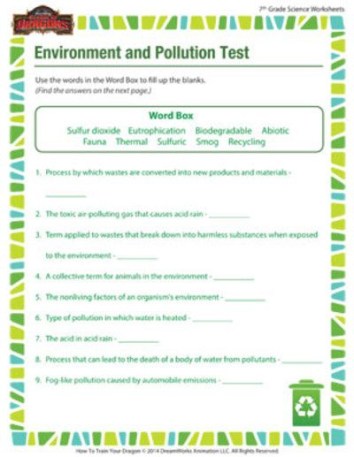
When working with files, it's crucial to handle potential errors that may occur during the reading process. Here are some common error scenarios and how to handle them:
- File Not Found: If the specified file doesn't exist, the program should gracefully handle this error. You can use the
is_open
function to check if the file was successfully opened. If not, display an appropriate error message and exit the program. - Invalid File Format: In some cases, the file format might be unexpected or invalid. For example, if you're expecting a specific data format but encounter an unexpected line, you should handle this error. You can use conditional checks to validate the data and take appropriate actions.
- File I/O Errors: While reading data from the file, I/O errors might occur due to various reasons, such as disk errors or unexpected conditions. You can use the
ferror
function to check for errors when using file I/O functions. If an error occurs, handle it by displaying an error message and taking necessary actions.
Best Practices and Tips
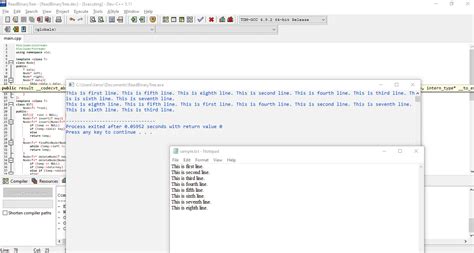
Here are some best practices and tips to keep in mind when reading data from files in C++:
- Use Appropriate Data Types: Choose the right data types to store the data you're reading. For example, use
int
for integers,double
for floating-point numbers, andstring
for textual data. - Handle File Open and Close: Always remember to check if the file was successfully opened and properly close it when you're done. This ensures that resources are managed efficiently and avoids potential issues.
- Validate Input Data: It's good practice to validate the data you're reading from the file. Check for unexpected values, incorrect formats, or missing data. This helps prevent errors and ensures the integrity of your program.
- Use Exception Handling: Consider using exception handling to gracefully handle errors during file reading. This allows you to catch and handle specific exceptions, making your code more robust and easier to maintain.
- Consider File Size: Be mindful of the file size you're working with. Large files might require additional memory or processing power. Plan your file reading strategy accordingly, especially if you're dealing with big datasets.
By following these best practices and tips, you can ensure that your file reading operations in C++ are efficient, reliable, and error-free.
Conclusion

Reading data from a file is a fundamental task in C++ programming, and understanding the various methods available is crucial for effective file handling. In this blog post, we explored three primary approaches: using the ifstream
class, file I/O functions, and C++ streams. Each method has its advantages and use cases, and choosing the right one depends on your specific requirements and preferences.
Whether you're reading line by line, individual characters, or specific data types, the techniques outlined in this post will help you accomplish your file reading tasks efficiently. Remember to handle errors gracefully, validate input data, and follow best practices to ensure the reliability and performance of your C++ programs.
How do I read data from a file in C++ using the ifstream class?
+To read data from a file using the ifstream class, you need to include the necessary header
What are the file I/O functions in C++ for reading data from a file?
+The file I/O functions in C++ for reading data from a file include fopen, fgets, fgetc, fscanf, and fclose. These functions provide a more low-level approach to file handling and are part of the cstdio library.
How can I use C++ streams to read data from a file?
+To use C++ streams to read data from a file, you need to include the necessary header