Matlab Visualize Triangular Mesh
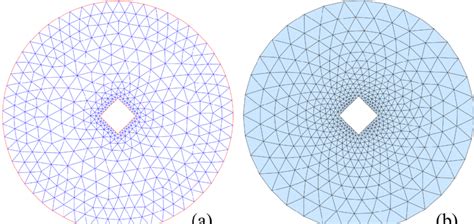
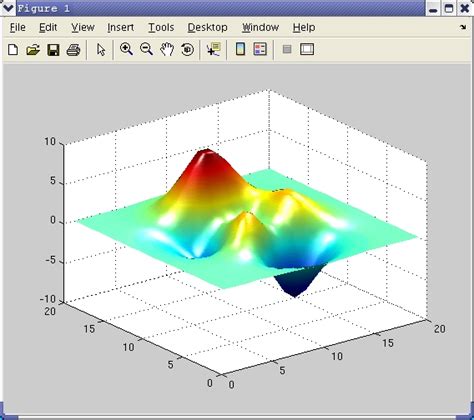
Introduction to Triangular Mesh Visualization in Matlab
Matlab provides a comprehensive set of tools for visualizing and analyzing data, including the ability to create and manipulate triangular meshes. A triangular mesh is a collection of triangles that are used to approximate the surface of a 3D object. In this post, we will explore how to visualize triangular meshes in Matlab, including how to create, manipulate, and customize the visualization.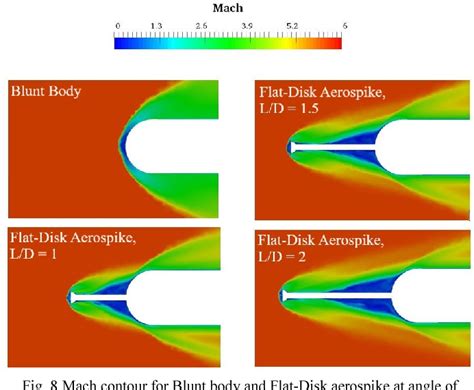
Creating a Triangular Mesh in Matlab
To create a triangular mesh in Matlab, you can use the trimesh function, which takes the vertices and faces of the mesh as input. The vertices are defined by a set of 3D coordinates, and the faces are defined by a set of indices that reference the vertices. For example:% Define the vertices
vertices = [-1 -1 0; 1 -1 0; 1 1 0; -1 1 0; 0 0 1];
% Define the faces
faces = [1 2 5; 2 3 5; 3 4 5; 4 1 5];
% Create the triangular mesh
trimesh(faces, vertices);
This code will create a simple triangular mesh with five vertices and four faces.
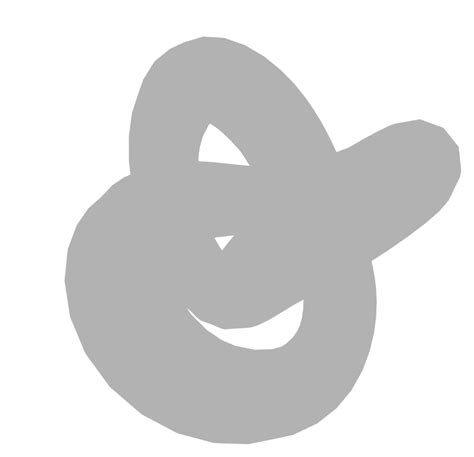
Customizing the Visualization
Once you have created the triangular mesh, you can customize the visualization using various options available in the trimesh function. For example, you can change the color of the mesh, add lighting effects, or modify the camera view. Here are a few examples:% Change the color of the mesh
trimesh(faces, vertices, 'EdgeColor', 'red');
% Add lighting effects
light;
lighting gouraud;
% Modify the camera view
view(45, 30);
You can also use other functions, such as axis, xlabel, ylabel, and zlabel, to customize the axes and add labels to the visualization.
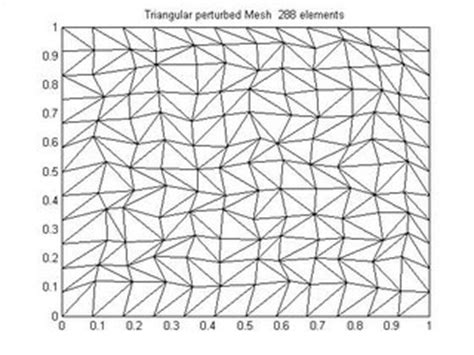
Working with Complex Meshes
When working with complex meshes, it can be useful to use other functions, such as patch or surf, to visualize the mesh. These functions can be used to create more complex visualizations, such as a mesh with multiple colors or a mesh with a texture. For example:% Create a mesh with multiple colors
c = ones(size(faces, 1), 1);
for i = 1:size(faces, 1)
if mod(i, 2) == 0
c(i) = 0.5;
end
end
trimesh(faces, vertices, 'EdgeColor', 'interp', 'FaceVertexCData', c);
This code will create a mesh with alternating colors.
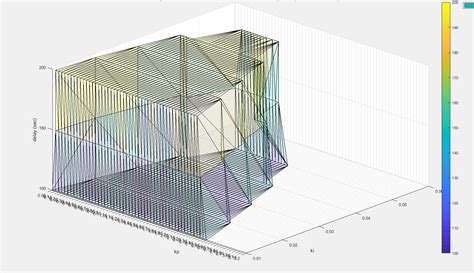
Using Matlab’s Built-in Mesh Functions
Matlab provides a range of built-in functions for working with meshes, including functions for creating, manipulating, and visualizing meshes. Some of these functions include: * trimesh: creates a triangular mesh from a set of vertices and faces * trimesh with options: customizes the visualization of the mesh * patch: creates a patch mesh from a set of vertices and faces * surf: creates a surface mesh from a set of vertices and faces * mesh: creates a mesh from a set of vertices and faces * delaunay: creates a Delaunay triangulation from a set of points * voronoi: creates a Voronoi diagram from a set of pointsHere is an example table showing some of the built-in mesh functions in Matlab:
Function | Description |
---|---|
trimesh | Creates a triangular mesh from a set of vertices and faces |
patch | Creates a patch mesh from a set of vertices and faces |
surf | Creates a surface mesh from a set of vertices and faces |
mesh | Creates a mesh from a set of vertices and faces |
delaunay | Creates a Delaunay triangulation from a set of points |
voronoi | Creates a Voronoi diagram from a set of points |
💡 Note: The choice of function will depend on the specific application and the type of mesh being used.
In summary, Matlab provides a range of tools for visualizing and analyzing triangular meshes, including the ability to create and manipulate meshes, customize the visualization, and use built-in mesh functions.
The key points to take away are the ability to create a triangular mesh using the trimesh function, customize the visualization using various options, and use other functions, such as patch or surf, to create more complex visualizations. Additionally, Matlab’s built-in mesh functions provide a range of tools for working with meshes, including functions for creating, manipulating, and visualizing meshes.
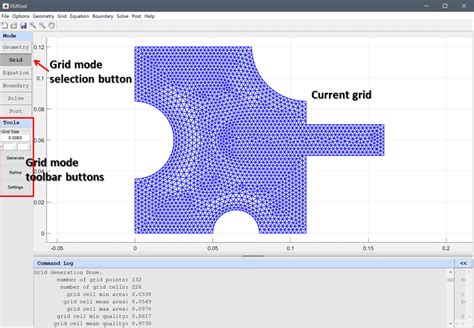
What is a triangular mesh?
+A triangular mesh is a collection of triangles that are used to approximate the surface of a 3D object.
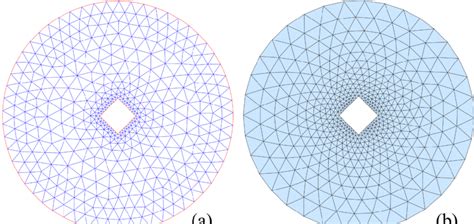
How do I create a triangular mesh in Matlab?
+You can create a triangular mesh in Matlab using the trimesh function, which takes the vertices and faces of the mesh as input.
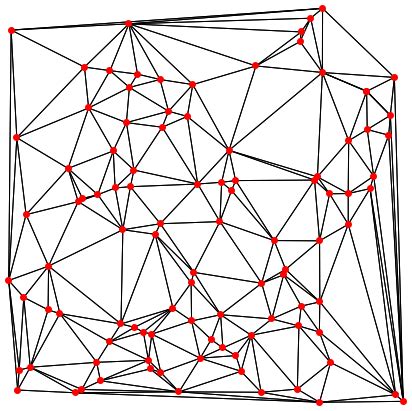
What are some common applications of triangular meshes?
+Triangular meshes are commonly used in computer-aided design (CAD), computer-generated imagery (CGI), and video games, as well as in scientific simulations, such as finite element analysis.